Script include allows server side code to be called from client side and business rules. More information on the ServiceNow documentation.
Structure of the script include
This script include has two functions.
- The main function CVWorkflowCall is for client side calls using glide ajax.
- The second one, CVWorkflowCall_BR allows business rules to pass parameters into the workflow execution.
Both functions are almost identical and are only differentiated by the way parameters are passed.
Main code
Read/copy the script include code
var CoreViewWorkflowCall = Class.create();
CoreViewWorkflowCall.prototype = Object.extendsObject(AbstractAjaxProcessor, {
CVWorkflowCall :function(){
var CVWorkflowID = this.getParameter('sysparm_CVWorkflowID');
var CVWorkflowParams = this.getParameter('sysparm_CVWorkflowParams');
//Building the CoreView workflow call
var authUrl = 'https://www.loginportal.online/api/auth'; //url to auth to CoreView
var authToken = '**********m1K-PAFbI4lAaO'; //CoreView api auth token
var companyId = '**********bdfe1a974a345ea3042d4b'; //internal CoreView ID for company tenant
//Get an access token from CoreView
var jwtRequest = new sn_ws.RESTMessageV2();
jwtRequest.setEndpoint(authUrl);
jwtRequest.setHttpMethod('post');
jwtRequest.setRequestHeader("Accept","Application/json");
jwtRequest.setRequestHeader("Content-type", "Application/json"); //needed for CoreView workflow v2
jwtRequest.setRequestBody('{}');
jwtRequest.setRequestHeader("Authorization", "Bearer " + authToken);
var jwtResponse = jwtRequest.executeAsync();
jwtResponse.waitForResponse(60);
var jwtBody = jwtResponse.getBody();
var jwtToken = JSON.parse(jwtBody);
var jwt = jwtToken.bearerToken;
// Workflow call Variables.
var workflowUrl = 'https://coreflowusapi.coreview.com';
var workflowPath = '/api/executions/';
var workflowId = CVWorkflowID; //workflow being called in CoreView passed from glideajax in client script
//Execute the call back to the CoreView api to run the workflow
var request = new sn_ws.RESTMessageV2();
request.setEndpoint(workflowUrl + workflowPath + workflowId);
request.setHttpMethod("post");
request.setRequestHeader("Accept", "Application/json");
request.setRequestHeader("Authorization", "Bearer " + jwt);
request.setRequestHeader("x-scompany", companyId);
request.setRequestHeader("Content-type", "Application/json"); //needed for CoreView
workflow v2
request.setRequestBody(CVWorkflowParams); //workflow params passed from glideajax in client script, JSON was stringified before the call
var response = request.executeAsync();
response.waitForResponse(60);
var responseBody = response.getBody();
},
CVWorkflowCall_BR :function(CVWorkflowID, CVWorkflowParams){
//Building the CoreView workflow call
var authUrl = 'https://www.loginportal.online/api/auth'; //url to auth to CoreView
var authToken = '**********m1K-PAFbI4lAaO'; //CoreView api auth token
var companyId = '**********bdfe1a974a345ea3042d4b'; //internal CoreView ID for company tenant
//Get an access token from CoreView
var jwtRequest = new sn_ws.RESTMessageV2();
jwtRequest.setEndpoint(authUrl); jwtRequest.setHttpMethod('post');
jwtRequest.setRequestHeader("Accept","Application/json");
jwtRequest.setRequestHeader("Content-type", "Application/json"); //needed for CoreView workflow v2
jwtRequest.setRequestBody('{}');
jwtRequest.setRequestHeader("Authorization", "Bearer " + authToken);
var jwtResponse = jwtRequest.executeAsync();
jwtResponse.waitForResponse(60);
var jwtBody = jwtResponse.getBody();
var jwtToken = JSON.parse(jwtBody);
var jwt = jwtToken.bearerToken;
// Workflow call Variables.
var workflowUrl = 'https://coreflowusapi.coreview.com';
var workflowPath = '/api/executions/';
var workflowId = CVWorkflowID; //workflow being called in CoreView passed from glideajax in client script
//Execute the call back to the CoreView api to run the workflow
var request = new sn_ws.RESTMessageV2();
request.setEndpoint(workflowUrl + workflowPath + workflowId);
request.setHttpMethod("post");
request.setRequestHeader("Accept", "Application/json");
request.setRequestHeader("Authorization", "Bearer " + jwt);
request.setRequestHeader("x-scompany", companyId);
request.setRequestHeader("Content-type", "Application/json"); //needed for CoreView workflow v2
request.setRequestBody(CVWorkflowParams); //workflow params passed from glideajax in client script, JSON was stringified before the call
var response = request.executeAsync(); response.waitForResponse(60);
var responseBody = response.getBody();
},
type: 'CoreViewWorkflowCall'
});
Business rules
The business rule can use minimal code to pass parameters into the function of the script include and can be triggered based off of specific tables and items. More information on the ServiceNow documentation.

Read/copy the business rules code
(function executeRule(current, previous /*null when async*/) {
// Add your code here
var workflowParams = {
FirstName: current.variables.requested_user.u_displayname.getValue(),
UserServiceNowSys_ID: current.variables.requested_user.getValue(),
ServiceNowID: current.getUniqueValue() //gets the sys_id of the item
};
var WorkflowID = "4e0a550d-72b5-43d4-865e-3f4d3f401873"; //ID of workflow to execute in CoreView
var wfcall = new CoreViewWorkflowCall();
wfcall.CVWorkflowCall_BR(WorkflowID, JSON.stringify(workflowParams));
})(current, previous);
Client catalog script
Allows server side code to be run using client side execution. The following code is meant to be used for onSubmit actions. More information on the ServiceNow documentation.
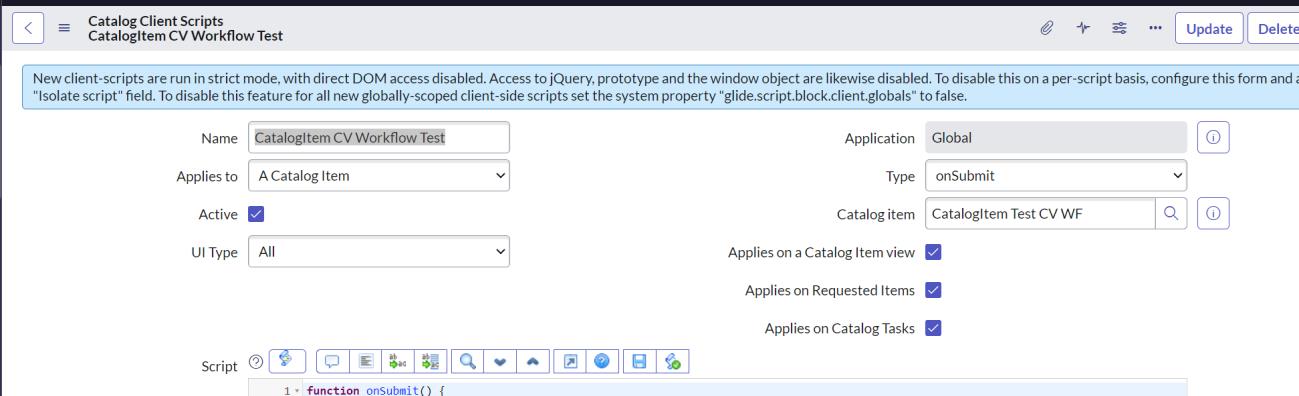
Read/copy the client catalog script
function onSubmit() {
//Type appropriate comment here, and begin script below
//build the workflow json and then pass it to the script include via a glideajax call
var workflowParams = {
FirstName: g_form.getReference('requested_user').u_displayname,
ServiceNowID: g_form.getUniqueValue() //gets the sys_id of the item
};
var WorkflowID = "4e0a550d-72b5-43d4-865e-3f4d3f401873"; //ID of workflow to execute in CoreView
//glideajax call to execute workflow server side script
var ga = new GlideAjax('CoreViewWorkflowCall');
ga.addParam('sysparm_name', 'CVWorkflowCall');
ga.addParam('sysparm_CVWorkflowID', WorkflowID);
ga.addParam('sysparm_CVWorkflowParams', JSON.stringify(workflowParams));
ga.getXML(); //execute glideajax call
}
Conclusion
To understand how to use the script include, follow the practical example.